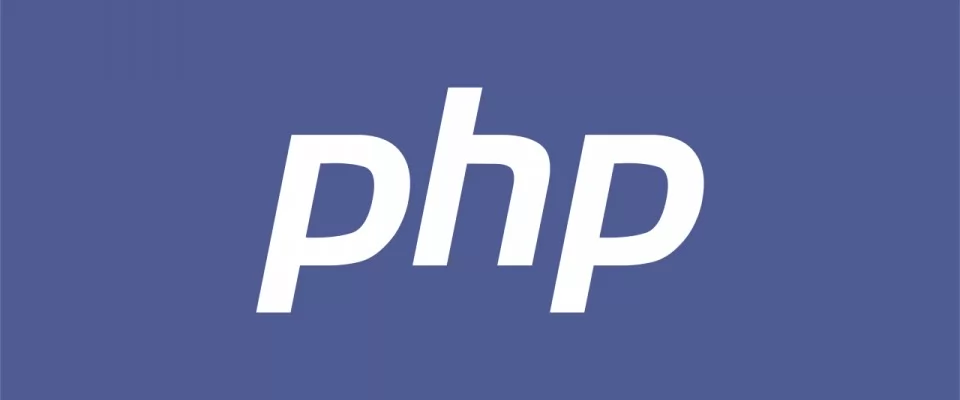
Mastering the essentials: A deep dive into PHP s top functionalities
PHP never ceases to surprise me with its built-in capabilities. These are a few of the functions I find most fascinating.
1. Levenshtein
This function uses the Levenshtein algorithm to calculate the disparity or "distance" between two text strings. Named after its creator, Vladimir Levenshtein, it measures how identical two words or sentences are.
For instance:
levenshtein("PHP is wonderful", "PHP is wonderful"); // Returns 0 levenshtein("Bright themes", "are great"); // Returns 13
The greater the disparity, the greater the distance.
2. Easter Dates
Would you believe PHP can tell you when Easter is for any given year? Easter's date is determined based on lunar and equatorial events. Here's a quick check for 2023:
date('Y-m-d', easter_date(2023)); // Outputs 2023-04-08
3. Forks
Async capabilities in PHP? Yes! The CLI version of PHP introduces us to the pcntl functions, notably the pcntl_fork. It lets you produce and supervise multiple PHP processes.
A snippet demonstrating its asynchronous potential:
function async(Process $process): Process { // ... (provided code) }
For ease of use, I developed a package: spatie/async.
4. Metaphone
Much like levenshtein, metaphone crafts a phonetic version of a string:
metaphone("Bright theme colors!"); // Outputs LFTKLRSXMS
5. Built-in DNS
PHP can interpret DNS using the
dns_get_record function
. It fetches DNS details, as the name suggests.
dns_get_record("exampledomain.com"); { ["host"] => "exampledomain.com", ["class"] => "IN", ["ttl"] => 7200, ["type"] => "A", ["ip"] => "192.0.2.1" }
n this example, querying the DNS record for "exampledomain.com" provides details like its IP address, Time-To-Live (TTL), and so on.
6. Recursive Array Merging
I'm including array_merge_recursive
here because I used to misinterpret its utility. Contrary to my initial belief, it's not just for merging multi-tiered arrays!
Certainly! Here's a rephrased and similar example: ```php $primary = [ 'tag' => 'initial' ]; $alternate = [ 'tag' => 'replacement' ]; array_merge_recursive($primary, $alternate); { ["tag"] => { "initial", "replacement", } } ```
In this case, we're merging two arrays containing the 'tag' key, and `array_merge_recursive` combines their values into a nested array.
7. Mail
Yes, PHP has a built-in function to dispatch emails. While I personally wouldn't use it for critical tasks, it's handy:
mail($to, $subject, $message);
8. DL
In PHP, there exists a function allowing on-the-fly extension loading: dl. If an extension isn't already loaded, this function can pull it in.
if (!extension_loaded('sampleext')) { if (strtoupper(substr(PHP_OS, 0, 3)) === 'WIN') { dl('php_sampleext.dll'); } else { dl('sampleext.so'); } }
In this code, we're checking if a fictional extension named 'sampleext' is loaded. If not, it will attempt to dynamically load the appropriate shared library based on the operating system.
9. Glob
One of the most practical functions, glob, retrieves paths that match a given pattern:
glob(__DIR__ . '/data/articles/*.md'); glob(__DIR__ . '/data/*/*.md'); { /route/to/data/articles/story.md, /route/to/data/topics/tale.md, … }
In this version, we're using the glob function to search for Markdown files in a hypothetical directory structure related to articles and topics.
10. Sun Info
Did you know PHP can also predict sunrises and sunsets for any given date? You'd need to provide the geographical coordinates for accurate results.
That's just the tip of the iceberg when it comes to PHP's potential!
date_sun_info( timestamp: strtotime('2023-02-15'), latitude: 51.5074, longitude: 0.1278, ) { ["sunrise"] => 1675500000 ["sunset"] => 1675540000 ["transit"] => 1675525000 ["civil_twilight_begin"] => 1675495000 ["civil_twilight_end"] => 1675545000 ["nautical_twilight_begin"] => 1675490000 ["nautical_twilight_end"] => 1675550000 ["astronomical_twilight_begin"] => 1675485000 ["astronomical_twilight_end"] => 1675555000 }
In this variation, we're getting the sun-related information for London (latitude and longitude of London) on 15th February 2023. Note: The timestamps are fictional and just for illustrative purposes.
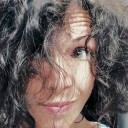
Silvia Mazzetta
Web Developer, Blogger, Creative Thinker, Social media enthusiast, Italian expat in Spain, mom of little 9 years old geek, founder of @manoweb. A strong conceptual and creative thinker who has a keen interest in all things relate to the Internet. A technically savvy web developer, who has multiple years of website design expertise behind her. She turns conceptual ideas into highly creative visual digital products.
Related Posts
How to Track Flight Status in real-time using the Flight Tracker API
The Flight Tracker API provides developers with the ability to access real-time flight status, which is extremely useful for integrating historical tracking or live queries of air traffic into your…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
PHP - The Singleton Pattern
The Singleton Pattern is one of the GoF (Gang of Four) Patterns. This particular pattern provides a method for limiting the number of instances of an object to just one.…
How to Send Email from an HTML Contact Form
In today’s article we will write about how to make a working form that upon hitting that submit button will be functional and send the email (to you as a…
The State of PHP 8: new features and changes
PHP 8.0 has been released last November 26: let's discover together the main innovations that the new version introduces in this language. PHP is one of the most popular programming languages…
HTTP Cookies: how they work and how to use them
Today we are going to write about the way to store data in a browser, why websites use cookies and how they work in detail. Continue reading to find out how…
The most popular Array Sorting Algorithms In PHP
There are many ways to sort an array in PHP, the easiest being to use the sort() function built into PHP. This sort function is quick but has it's limitations,…
MySQL 8.0 is now fully supported in PHP 7.4
MySQL and PHP is a love story that started long time ago. However the love story with MySQL 8.0 was a bit slower to start… but don’t worry it rules…
A roadmap to becoming a web developer in 2019
There are plenty of tutorials online, which won't cost you a cent. If you are sufficiently self-driven and interested, you have no difficulty training yourself. The point to learn coding…
10 PHP code snippets to work with dates
Here we have some set of Useful PHP Snippets, which are useful for PHP Developers. In this tutorial we'll show you the 10 PHP date snippets you can use on…
8 Free PHP Books to Read in Summer 2018
In this article, we've listed 8 free PHP books that can help you to learn new approaches to solving problems and keep your skill up to date. Practical PHP Testing This book…
Best Websites to Learn Coding Online
You know and we know that it’s totally possible to learn to code for free... If you can teach yourself how to write code, you gain a competitive edge over your…
